Thư viện tri thức trực tuyến
Kho tài liệu với 50,000+ tài liệu học thuật
© 2023 Siêu thị PDF - Kho tài liệu học thuật hàng đầu Việt Nam
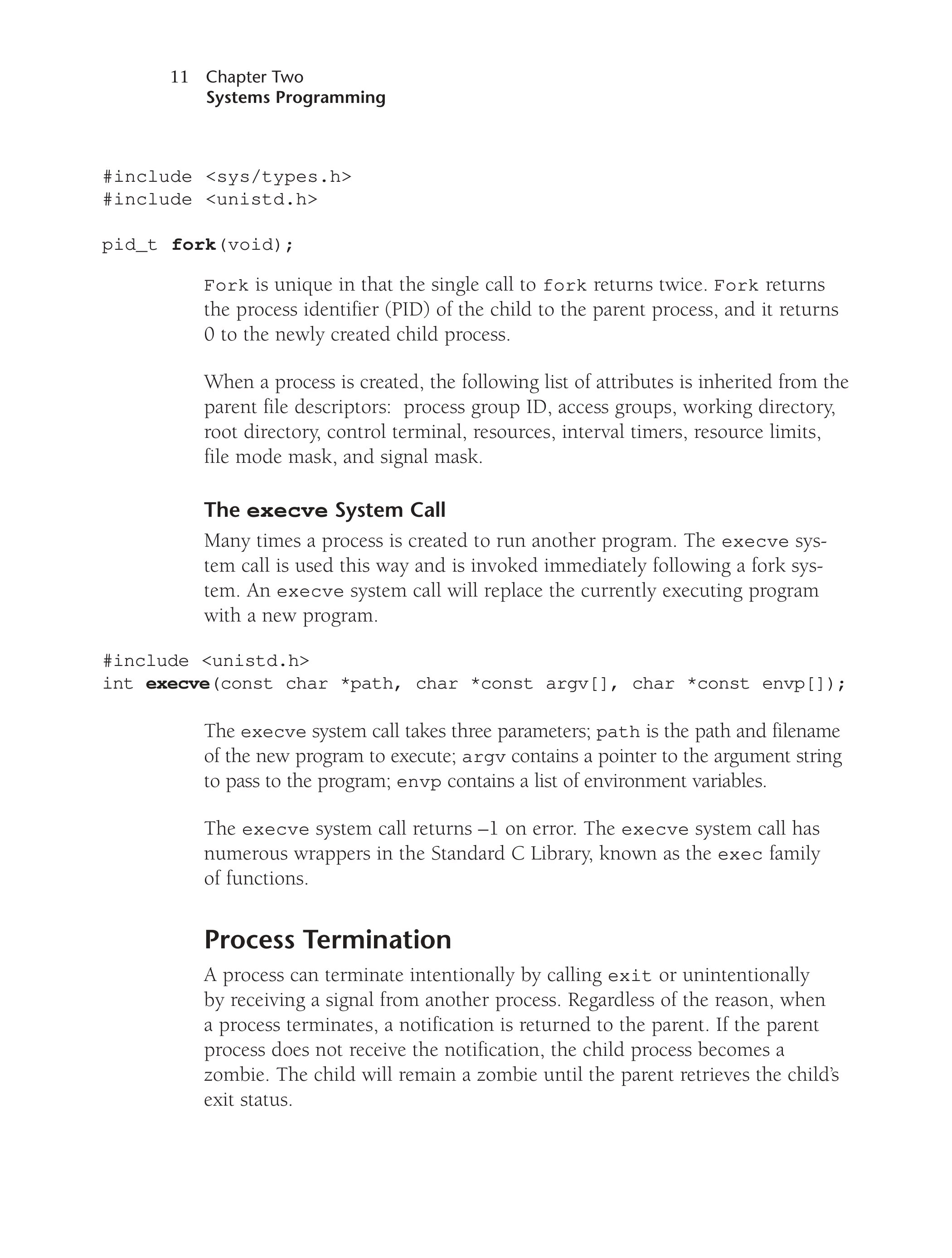
Embedded FreeBSD Cookbook phần 2 ppt
Nội dung xem thử
Mô tả chi tiết
11 Chapter Two
Systems Programming
#include <sys/types.h>
#include <unistd.h>
pid_t fork(void);
Fork is unique in that the single call to fork returns twice. Fork returns
the process identifier (PID) of the child to the parent process, and it returns
0 to the newly created child process.
When a process is created, the following list of attributes is inherited from the
parent file descriptors: process group ID, access groups, working directory,
root directory, control terminal, resources, interval timers, resource limits,
file mode mask, and signal mask.
The execve System Call
Many times a process is created to run another program. The execve system call is used this way and is invoked immediately following a fork system. An execve system call will replace the currently executing program
with a new program.
#include <unistd.h>
int execve(const char *path, char *const argv[], char *const envp[]);
The execve system call takes three parameters; path is the path and filename
of the new program to execute; argv contains a pointer to the argument string
to pass to the program; envp contains a list of environment variables.
The execve system call returns –1 on error. The execve system call has
numerous wrappers in the Standard C Library, known as the exec family
of functions.
Process Termination
A process can terminate intentionally by calling exit or unintentionally
by receiving a signal from another process. Regardless of the reason, when
a process terminates, a notification is returned to the parent. If the parent
process does not receive the notification, the child process becomes a
zombie. The child will remain a zombie until the parent retrieves the child’s
exit status.
12 Embedded FreeBSD
Cookbook
The _exit System Call
A process will terminate when the program invokes the _exit system call.
The _exit system call will cause the SIGCHLD signal to be thrown to the
parent process.
#include <unistd.h>
void _exit(int status);
The _exit system call will never return. The _exit system call is more
commonly called by the Standard C library function exit.
The wait System Call
The wait system call allows a parent process to check to see if termination
information is available for a child process. Wait will suspend execution
until the child process returns.
#include <sys/types.h>
#include <sys/wait.h>
pid_t wait(int *status);
On success, the child process identifier (PID) is returned to the parent process,
or –1 is returned if there is an error. The child’s exit status is returned by the
status parameter.
An Example
Listing 2-1 illustrates the usage of the fork, wait, execve, and exit calls.
The parent process makes a fork system call to create a child process.
There are two control paths in the main program, one for the parent and
another for the child.
int main(int argc, char **argv)
{
pid_t pid;
int status;
if ((pid = fork()) > 0)
{
printf(“%d: waiting for the child\n”, pid);
wait(&status);